MT5 Trailing Stop ExpertA trailing stop is nothing more than a stop loss that moves as price changes. For this standard trailing stop the rules are simple:No stop loss is applied if the stop loss is worse than the trade opening priceThe top loss only moves in a positive...
MT5 Trailing Stop Expert
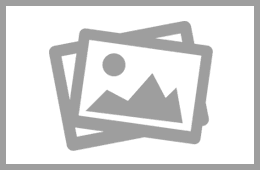
Categories:
trailing stoploss